Welcome to the 453 QT Designer Tutorial
Part 1 - Creating a basic QT project and adding some widgets.
- This is a shot of QT Designer where a project is being worked on. Notice the areas
labelled in red.
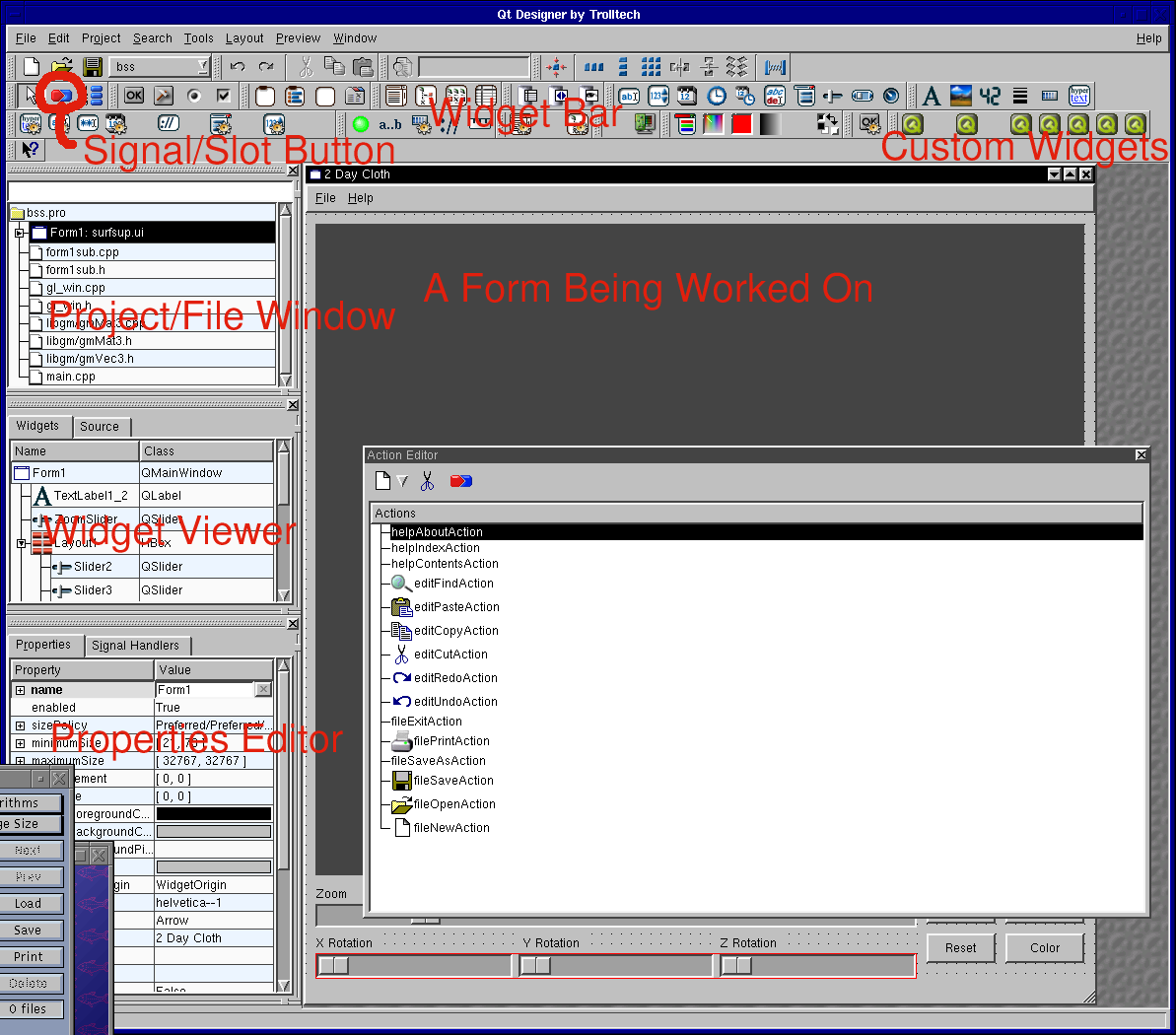
- Run QT Designer by typing "designer &" at the shell.
- Create a new QT project by going to File -> New -> C++ Project.
- Name the project file "easy.pro" and ensure it is saved in its own directory.
- Create your project's form by going to File -> New -> Dialog.
- This creates a form for you.
- Change the name of the form to EasyForm. This is an important step, because the
object representing the form will be called EasyForm. To change the name you click
on the form, so that the properties window contains the form's properties. Then
you scroll up or down along the properties window to find name. In the text box
next to this you replace "Form1" with "EasyForm".
- Change the caption of the form to whatever you want. Do this by looking for a changing
the caption property in the properties window.
- You should now have something like this.
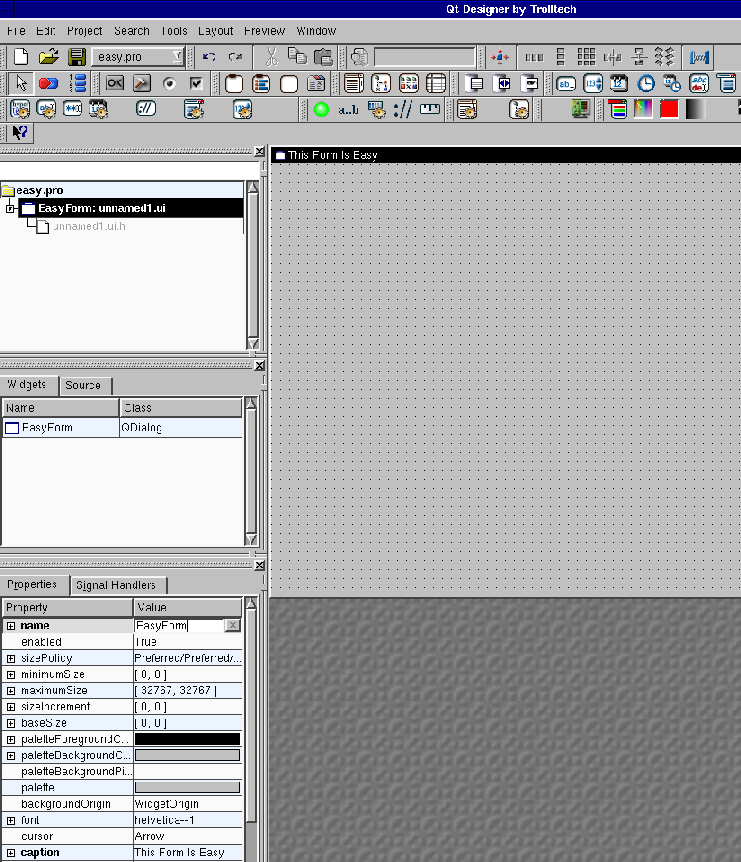
- Add some widgets. This is done by dragging the widgets from the widget bar to
where you'd like to place them on the form. When I refer to the widget bars I
am talking about the lowest two toolbars at the top of the window.
- Add three sliders (for rotation).
- Add another slider (for scaling/zooming).
- Add appropriate labels for the sliders.
- Add an LCD display (for showing the amount of scaling/zooming).
- Create a layout for the three rotation sliders. Do this by dragging a box
over the three sliders to highlight them, then right-click and select lay-out
horizontal or lay-out vertically depending on how you've arranged the
sliders.
- Rename the sliders appropriately (e.g., name the X rotation slider
xRotSlider).
- Event Handling. Connect the valueChanged(int) signal of the scale slider to the
display(int) slot of the LCD Number widget.
- Do this by clicking the pill shaped signal/slot button.

- Then left click on the zoom slider, and dragging over to the LCD Number
before releasing the mouse button.
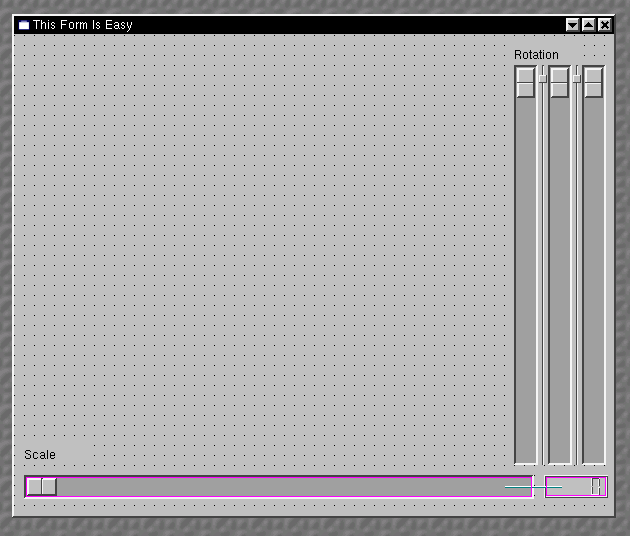
- This brings up the signal/slot dialog where you select the appropriate
signal and slot.
You should end up with this.
Then click the OK buttton.
- Save your work by going to File -> Save All.
- Create your main.cpp file by going to File -> New -> main.cpp.
- Ensure that the #include in main.cpp has been created correctly. In the Project
Overview window double click on main.cpp, this will bring up an editor with the
contents of main.cpp. Ensure the #include is including easyform.h rather than
unnamed.h or something similar.
- Save your work.
- In your shell, type "ls" and ensure that the file easy.pro and easyform.ui have been
created. If they haven't make certain you've saved your project and dialog to the
directory you're currently in. Then type qmake easy.pro. This creates a
makefile for you.
- Type make to compile your program.
- Type ./easy to run the program you've created.
- You should now see something like this!
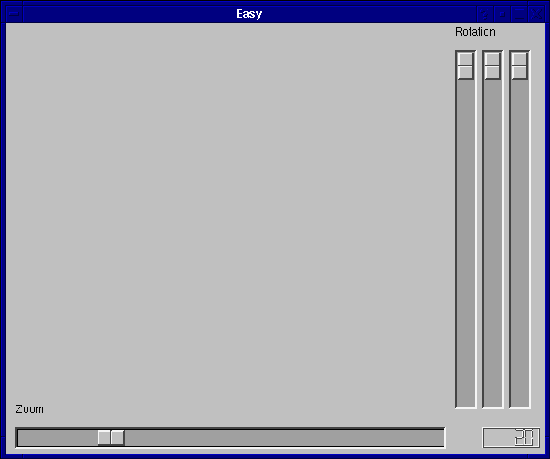
Check Point 1 - You should now have a running QT project with some sliders that don't
do much, except for the scaling slider that causes the LCD number to change. If not,
try again!
FYI
If you look around the directory you have created you will find the following files:
- Makefile - the file containing the compiling instructions for your program.
- easy - the executable you have created.
- easy.db - you can ignore this file.
- easy.pro - your project file.
- easyform.ui - this file contains information about the EasyForm dialog you've created.
- main.cpp - the main file we created earlier.
However if you do a "ls -al" you will find three hidden directories have also been created. They are:
- .moc - this is where the moc files and stored for each of your forms. This are generated by QT and you
should never have to edit them.
- .obj - this contains all of the .o files generated by the compiler and are linked to create your program.
- .ui - this directory contains .cpp and .h code for each of your forms. This files are autogenerated by QT
so don't bother changing them as your changes will be overwritten.
So basically you never have to touch anything in these hidden directories. This is why they are hidden.
Now continue on to Part 2!