OpenGL - 3D Shapes
Using the same #d cube files you have been working on, make changes to
view your first quadric. A sphere.
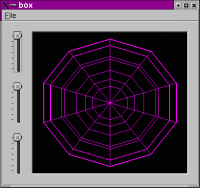
- Quadrics uses GLU to render 3D shapes such as spheres, disks,
and cylinders.
- In glbox.cpp (in paintGL):
- To create a quadric use:
GLUquadricObj* q = gluNewQuadric( );
- The default values are probably not what you want, so then
you have to specify the rendering attributes.
- This controls the orientation of the sphere either outside
to view from the outside, or inside to - can you guess?!
gluQuadricOrientation(q, GLU_OUTSIDE);
- If you wish to light your quadrics you need to specify
smooth shading.
gluQuadricNormals(q, GLU_SMOOTH);
- You can choose the drawing style of the quadric. Try
changing this value to see the effects of: GLU_POINT,
GLU_SILHOUETTE, GLU_FILL.
gluQuadricDrawStyle ( q, GLU_LINE );
- Finally draw the type of quadric. Try drawing gluCylinder(),
gluDisk(), or gluPartialDisk() as well.
gluSphere ( q, 1.8, 10, 10 );
- Remember you will have to delete the quadri when you are
finished. using:.
gluDeleteQuadric();
-
Remember to check the documentation.
Read chapter 3 of the OpenGL Programming Guide: Viewing. Pay special
attention to Matrices and how OpenGL deals with them.
Here is an updated and re-commented version of the files.
www.cpsc.ucalgary.ca/~pj/453/box/3DSphere/
Useful References
OpenGL Programming Guide
(The Red Book).
OpenGL Index in Alphabetic Order
(This is pretty long)
The OpenGL Website
The OpenGL Website - tutorials
Contact me
email: pj@cpsc.ucalgary.ca
Tel: (403) 220 7041.